C# Tutorial
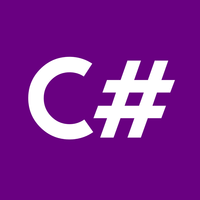
C# tutorial provides basic and advanced concepts of C#. Our C# tutorial is designed for beginners and professionals.
C# is a programming language of .Net Framework.
Our C# tutorial includes all topics of C# such as first example, control statements, objects and classes, inheritance, constructor, destructor, this, static, sealed, polymorphism, abstraction, abstract class, interface, namespace, encapsulation, properties, indexer, arrays, strings, regex, exception handling, multithreading, File IO, Collections etc.
What is C#
C# is pronounced as "C-Sharp". It is an object-oriented programming language provided by Microsoft that runs on .Net Framework.
By the help of C# programming language, we can develop different types of secured and robust applications:
- Window applications
- Web applications
- Distributed applications
- Web service applications
- Database applications etc.
C# is approved as a standard by ECMA and ISO. C# is designed for CLI (Common Language Infrastructure). CLI is a specification that describes executable code and runtime environment.
C# programming language is influenced by C++, Java, Eiffel, Modula-3, Pascal etc. languages.
C# Index
.Net Framework
C#
- C# Tutorial
- What is C#
- C++ vs C#
- Java vs C#
- C# History
- C# Features
- C# Example
- C# Variables
- C# Data Types
- C# Operators
- C# Keywords
C# Control Statements
C# Functions
C# Arrays
- C# Arrays
- C# Array to Function
- C# Multidimensional Array
- C# Jagged Arrays
- C# Params
- C# Array class
- C# Command Line Args
C# Objects and Classes
- C# Object and Class
- C# Constructor
- C# Destructor
- C# this
- C# static
- C# static class
- C# static constructor
- C# Structs
- C# Enum
C# Properties
C# Inheritance
C# Polymorphism
C# Abstraction
C# Namespace
C# Strings
C# String Functions
- String Clone()
- String Compare()
- String CompareOrdinal()
- String CompareTo()
- String Concat()
- String Contains()
- String Copy()
- String CopyTo()
- String EndsWith()
- String Equals()
- String Format()
- String GetEnumerator()
- String GetHashCode()
- String GetType()
- String GetTypeCode()
- String IndexOf()
- String Insert()
- String Intern()
- String IsInterned()
- String IsNormalized()
- String Normalize()
- String IsNullOrEmpty()
- IsNullOrWhiteSpace()
- String Join()
- String LastIndexOf()
- String LastIndexOfAny()
- String PadLeft()
- String PadRight()
- String Remove()
- String Replace()
- String Split()
- String StartsWith()
- String SubString()
- String ToCharArray()
- String ToLower()
- String ToLowerInvariant()
- String ToString()
- String ToUpper()
- String ToUpperInvariant()
- String Trim()
- String TrimEnd()
- String TrimStart()
C# Exceptions
- C# Exception Handling
- C# try/catch
- C# finally
- C# Custom Exception
- C# checked unchecked
- C# SystemException
C# File I/O
- C# FileStream
- C# StreamWriter
- C# StreamReader
- C# TextWriter
- C# TextReader
- C# BinaryWriter
- C# BinaryReader
- C# StringWriter
- C# StringReader
- C# FileInfo
- C# DirectoryInfo
- C# Serialization
- C# Deserialization
- C# System.IO
C# Collections
- C# Collections
- C# List<T>
- C# HashSet<T>
- C# SortedSet<T>
- C# Stack<T>
- C# Queue<T>
- C# LinkedList<T>
- C# Dictionary<K,V>
- C# SortedDictionary<K,V>
- C# SortedList<K,V>
C# Generics
C# Delegates
C# Reflection
C# Anonymous Functions
C# Multithreading
- C# Multithreading
- C# Thread Life Cycle
- C# Thread class
- C# Main Thread
- C# Thread Example
- C# Thread Sleep
- C# Thread Abort
- C# Thread Join
- C# Thread Name
- C# ThreadPriority
C# Synchronization
C# New Features
C# 2.0
- Partial types
- Iterators
- Nullable types
- Delegate Covariance
- Delegate inference
- Static classes
- Method group conversions (delegates)
C# 3.0
- Anonymous types
- Extension methods
- Query expression
- Partial method
- Implicitly typed local variables
- Object and collection initializers
- Auto-Implemented properties
- Lambda expression
- Expression trees
C# 4.0
- Dynamic binding
- Named and optional arguments
- Generic co and contravariance
- Embedded interop types ("NoPIA")